iOS点击输入框时自动移动到键盘之上
2015-10-12 本文已影响3139人
summer朱光文
1. 概述
本文要实现的是在iOS上点击输入框后,如果输入框在键盘之下,那么将自动移动界面使得输入框在键盘之上!就像Android的效果那样。效果图如下:
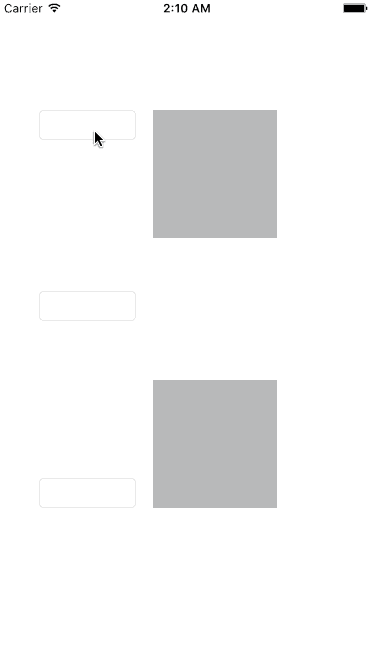
2. 一分钟实现该效果:
- 点击这里下载
WPAutoSpringTextViewController.h
,WPAutoSpringTextViewController.m
,UIResponder+FirstResponder.h
,UIResponder+FirstResponder.m
四个文件到你的工程中 - 修改你的ViewController的父类为WPAutoSpringTextViewController
大功告成,是不是很简单!如果想了解实现方法,请继续阅读。
3. 实现原理
首先,我们需要监听键盘的广播:
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(wpKeyboardWillShow:) name:UIKeyboardWillShowNotification object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(wpKeyboardWillHide:) name:UIKeyboardWillHideNotification object:nil];
键盘弹出广播中我们需要获取键盘弹出的高度,键盘弹出的时间。
float duration = [note.userInfo[UIKeyboardAnimationDurationUserInfoKey] floatValue];
CGFloat keyboardHeight = [note.userInfo[UIKeyboardFrameEndUserInfoKey] CGRectValue].size.height;
然后我们判断此时view需要移动的距离,这里首先获取firstResponder,然后判断其是否为UITextView或UITextField类或其子类,再根据该输入框在屏幕上的位置计算是否需要弹起view,需要则返回需要弹起的高度,不需要则为0。
- (CGFloat)shouldScrollWithKeyboardHeight:(CGFloat)keyboardHeight{
id responder = [UIResponder currentFirstResponder];
if([responder isKindOfClass:[UITextView class]] || [responder isKindOfClass:[UITextField class]]){
UIView *view = responder;
CGFloat y = [responder convertPoint:CGPointZero toView:[UIApplication sharedApplication].keyWindow].y;
CGFloat bottom = y + view.frame.size.height;
if(bottom > SCREEN_HEIGHT - keyboardHeight){
return bottom - (SCREEN_HEIGHT - keyboardHeight);
}
}
return 0;
}
最后当我们发现需要弹起页面时则动画弹起页面:
CGFloat shouldScrollHeight = [self shouldScrollWithKeyboardHeight:keyboardHeight];
if(shouldScrollHeight == 0){
return;
}
__weak WPAutoSpringTextViewController *weakSelf = self;
[UIView animateWithDuration:duration animations:^{
CGRect bounds = weakSelf.view.bounds;
weakSelf.view.bounds = CGRectMake(0, shouldScrollHeight + 10, bounds.size.width, bounds.size.height);
}];
这里主要的逻辑都已经完成了,点击在键盘覆盖范围内的输入框时,界面可以自动弹起。当点击空白区域时,键盘自动收起,这个功能代码如下:
self.view.userInteractionEnabled = YES;
[self.view addGestureRecognizer:[[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(viewClicked)]];
- (void)viewClicked{
if(keyboardIsShowing){
id responder = [UIResponder currentFirstResponder];
if([responder isKindOfClass:[UITextView class]] || [responder isKindOfClass:[UITextField class]]){
UIView *view = responder;
[view resignFirstResponder];
}
}
}
好了大功告成! 完整的代码如下。Github地址
https://github.com/MRsummer/WPAutoSpringKeyboard
#import "WPAutoSpringTextViewController.h"
#import "UIResponder+FirstResponder.h"
#define SCREEN_HEIGHT [UIScreen mainScreen].bounds.size.height
@implementation WPAutoSpringTextViewController{
BOOL keyboardIsShowing;
}
-(void)viewDidLoad{
[super viewDidLoad];
[self enableEditTextScroll];
self.view.userInteractionEnabled = YES;
[self.view addGestureRecognizer:[[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(viewClicked)]];
}
- (void)viewClicked{
if(keyboardIsShowing){
id responder = [UIResponder currentFirstResponder];
if([responder isKindOfClass:[UITextView class]] || [responder isKindOfClass:[UITextField class]]){
UIView *view = responder;
[view resignFirstResponder];
}
}
}
- (CGFloat)shouldScrollWithKeyboardHeight:(CGFloat)keyboardHeight{
id responder = [UIResponder currentFirstResponder];
if([responder isKindOfClass:[UITextView class]] || [responder isKindOfClass:[UITextField class]]){
UIView *view = responder;
CGFloat y = [responder convertPoint:CGPointZero toView:[UIApplication sharedApplication].keyWindow].y;
CGFloat bottom = y + view.frame.size.height;
NSLog(@"shouldScrollWithKeyboardHeight -->keyboradHeight %@, keyboradBottom %@, viewY %@, bottom %@", @(keyboardHeight), @(SCREEN_HEIGHT - keyboardHeight), @(y), @(bottom));
if(bottom > SCREEN_HEIGHT - keyboardHeight){
return bottom - (SCREEN_HEIGHT - keyboardHeight);
}
}
return 0;
}
- (void)enableEditTextScroll{
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(wpKeyboardWillShow:) name:UIKeyboardWillShowNotification object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(wpKeyboardWillHide:) name:UIKeyboardWillHideNotification object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(wpKeyboardDidShow) name:UIKeyboardDidShowNotification object:nil];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(wpKeyboardDidHide) name:UIKeyboardDidHideNotification object:nil];
}
- (void)wpKeyboardDidShow{
keyboardIsShowing = YES;
}
- (void)wpKeyboardDidHide{
keyboardIsShowing = NO;
}
- (void)wpKeyboardWillHide:(NSNotification *)note {
float duration = [note.userInfo[UIKeyboardAnimationDurationUserInfoKey] floatValue];
__weak WPAutoSpringTextViewController *weakSelf = self;
[UIView animateWithDuration:duration animations:^{
CGRect bounds = weakSelf.view.bounds;
weakSelf.view.bounds = CGRectMake(0, 0, bounds.size.width, bounds.size.height);
}];
}
- (void)wpKeyboardWillShow:(NSNotification *)note {
float duration = [note.userInfo[UIKeyboardAnimationDurationUserInfoKey] floatValue];
CGFloat keyboardHeight = [note.userInfo[UIKeyboardFrameEndUserInfoKey] CGRectValue].size.height;
CGFloat shouldScrollHeight = [self shouldScrollWithKeyboardHeight:keyboardHeight];
if(shouldScrollHeight == 0){
return;
}
__weak WPAutoSpringTextViewController *weakSelf = self;
[UIView animateWithDuration:duration animations:^{
CGRect bounds = weakSelf.view.bounds;
weakSelf.view.bounds = CGRectMake(0, shouldScrollHeight + 10, bounds.size.width, bounds.size.height);
}];
}
@end